General Information
Obtaining an API Key
You can generate an API key to access the Tegro.money REST service by visiting the store settings page.
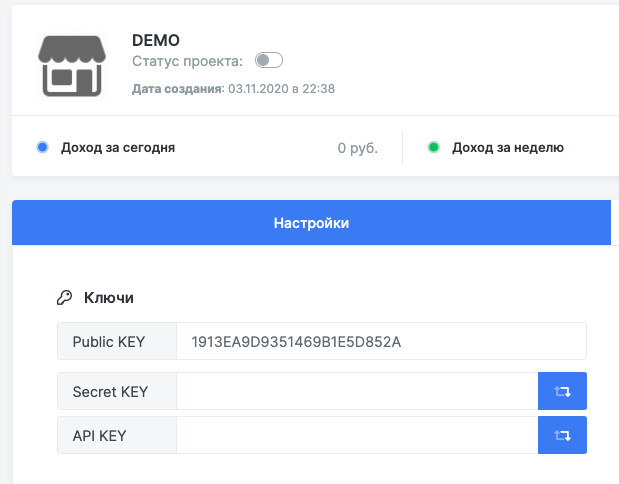
All data sent in requests to the Tegro.money service is done using the POST method over the HTTP protocol at the address https://tegro.money/api/method. Message parameters are organized as a JSON object.
You must include a signature along with the request. The entire request body needs to be signed exactly as it's sent to the Bank's server (after converting the request body into JSON for HTTP transmission).
In every request, the nonce parameter must be unique compared to the previous one! For instance, you can use the current time in seconds.
Utilize your secret key to create the signature. Construct the signature using the SHA-256 algorithm.
<?php
$api_key = 'EEFA1913EA9D9351469B1E5D852A';
$data = array(
'shop_id' => '1913EA9D9351469B1E5D852A',
'nonce' => time(),
);
$body = json_encode($data);
$sign = hash_hmac('sha256', $body, $api_key);
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://tegro.money/api/orders/",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => $body,
CURLOPT_HTTPHEADER => array(
"Authorization: Bearer $sign",
"Content-Type: application/json"
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
?>